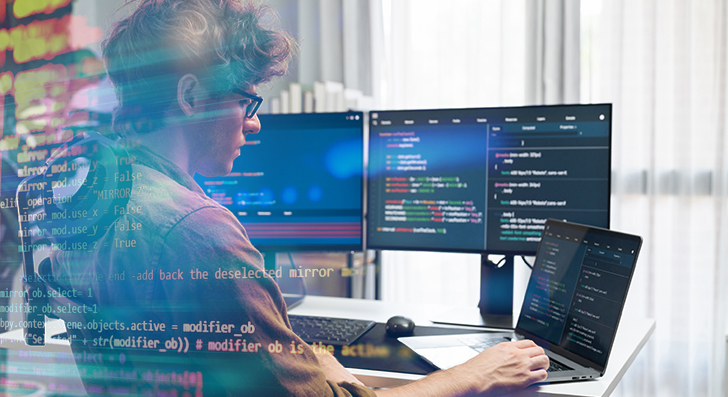
Scalability means your application can deal with growth—additional end users, a lot more information, and more targeted visitors—without the need of breaking. Being a developer, creating with scalability in your mind saves time and stress afterwards. Right here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it should be portion of your system from the beginning. Quite a few applications fall short once they improve quick for the reason that the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your procedure will behave under pressure.
Start out by creating your architecture being adaptable. Stay away from monolithic codebases wherever every thing is tightly linked. In its place, use modular design and style or microservices. These designs crack your app into more compact, unbiased parts. Each and every module or assistance can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it have to have to handle 1,000,000 end users or simply just 100? Choose the correct sort—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, even if you don’t want them still.
A further important point is to prevent hardcoding assumptions. Don’t compose code that only operates beneath latest ailments. Give thought to what would materialize If the person foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or event-driven techniques. These aid your app deal with much more requests with out obtaining overloaded.
Whenever you build with scalability in your mind, you're not just making ready for fulfillment—you happen to be minimizing foreseeable future head aches. A nicely-planned procedure is simpler to keep up, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Picking out the proper database is usually a essential Component of constructing scalable programs. Not all databases are built a similar, and using the Incorrect you can sluggish you down or perhaps induce failures as your application grows.
Start off by knowing your data. Could it be very structured, like rows inside of a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective fit. These are definitely potent with associations, transactions, and regularity. Additionally they aid scaling tactics like read replicas, indexing, and partitioning to manage much more website traffic and info.
In the event your details is more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with substantial volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more conveniently.
Also, think about your examine and write designs. Are you presently performing numerous reads with much less writes? Use caching and browse replicas. Are you presently handling a weighty generate load? Consider databases that could tackle high compose throughput, or maybe party-dependent details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also good to think ahead. You may not need to have State-of-the-art scaling options now, but choosing a databases that supports them suggests you received’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your entry designs. And always keep track of database overall performance as you expand.
In brief, the correct database is determined by your app’s construction, speed desires, And just how you assume it to increase. Just take time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is essential to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Start out by composing thoroughly clean, easy code. Stay clear of repeating logic and take away anything at all pointless. Don’t choose the most advanced Resolution if a simple 1 is effective. Maintain your functions shorter, centered, and easy to check. Use profiling equipment to find bottlenecks—destinations in which your code takes far too very long to run or takes advantage of excessive memory.
Subsequent, evaluate your databases queries. These often sluggish issues down in excess of the code itself. Ensure that Each and every query only asks for the information you truly need to have. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And stay away from executing too many joins, Specially across substantial tables.
If you observe a similar info staying asked for repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Make sure to check with massive datasets. Code and queries that get the job done great with 100 records may well crash whenever they have to manage one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to take care of more users and much more site visitors. If anything goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching are available. Both of these equipment support maintain your app quick, steady, and scalable.
Load balancing spreads incoming site visitors across several servers. As opposed to 1 server performing all the work, the load balancer routes buyers to unique servers based upon availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details quickly so it may be reused quickly. When people request exactly the same information and facts yet again—like a product web site or simply a profile—you don’t ought to fetch it in the databases each and every time. You are able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
2. Shopper-side caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances speed, and can make your application a lot more economical.
Use caching for things that don’t transform generally. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective applications. With each other, they assist your application deal with far more buyers, remain rapid, and Recuperate from challenges. If you propose to develop, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you'll need equipment that allow your application grow effortlessly. That’s the place cloud platforms and containers are available. They offer you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess foreseeable future ability. When website traffic improves, you can incorporate far more assets with just a couple clicks or routinely working with car-scaling. When website traffic drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and stability instruments. You may center on making your application as opposed to handling infrastructure.
Containers are One more essential Device. A container packages your app and all the things it really should operate—code, libraries, options—into 1 unit. This can make it effortless to move your app concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the most popular Software for this.
Whenever your app uses various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate aspects of your app into services. You may update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container instruments implies you could scale rapidly, deploy easily, and Get well quickly when troubles happen. In order for you your app to increase without boundaries, get started applying these resources early. They help save time, decrease chance, and help you remain centered on building, not repairing.
Watch Every thing
In case you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your app is executing, location problems early, Gustavo Woltmann blog and make far better selections as your application grows. It’s a key Portion of making scalable units.
Begin by tracking standard metrics like CPU utilization, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this data.
Don’t just keep track of your servers—check your app much too. Keep an eye on how long it takes for customers to load webpages, how often mistakes take place, and in which they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your reaction time goes previously mentioned a limit or perhaps a services goes down, you need to get notified quickly. This will help you correct concerns quickly, frequently prior to users even notice.
Checking can be valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it again just before it leads to real problems.
As your app grows, visitors and details increase. Devoid of monitoring, you’ll miss indications of difficulty right until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking aids you keep the app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for massive companies. Even modest applications want a solid foundation. By building very carefully, optimizing sensibly, and using the appropriate applications, you'll be able to Establish apps that improve smoothly with no breaking stressed. Begin modest, think huge, and Make intelligent.